| "summary": "Awesome iOS 11 AppStore Card Views", |
| "description": "[](http://cocoadocs.org/docsets/Cards)\n[](http://cocoadocs.org/docsets/Cards)\n\n\n\nCards brings to XCode the card views you can see in the new iOS XI Appstore.\n\n## Getting Started\n\n### Storyboard\n- Go to **main.storyboard** and add a **blank UIView**\n- Open the **Identity Inspector** and type '**CardHighligth**' the '**class**' field\n- Switch to the **Attributes Inspector** and **configure** it as you like. \n\n### Code\n```swift\n// Aspect Ratio of 5:6 is preferred\nlet card = CardHighlight(frame: CGRect(x: 100, y: 100, width: 200, height: 240))\ncard.backgroundColor = UIColor(red: 0, green: 94/255, blue: 112/255, alpha: 1)\ncard.backgroundImage = UIImage(named: \"flBackground\") // Comment this for the first view\ncard.icon = UIImage(named: \"flappy\")\ncard.title = \"Welcome to XI Cards !\"\ncard.itemTitle = \"Flappy Bird\"\ncard.itemSubtitle = \"Flap That !\"\nview.addSubview(card)\n```\n\n\n\n## Prerequisites\n\n- **XCode 9.0** or newer\n- **Swift 4.0**\n\n## Installation\n\n### Cocoapods\n```\nuse_frameworks!\npod 'Cards'\n```\n### Manual\n- **Download** the repo\n- ⌘C ⌘V the **'Cards' folder** in your project\n- In your **Project's Info** go to '**Build Phases**'\n- Open '**Compile Sources**' and **add all the files** in the folder\n\n## Overview\n\n\n\n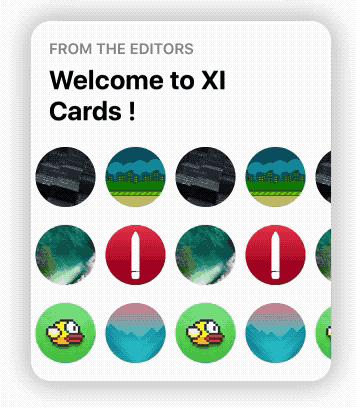\n\n\n## Customization\n\n```swift\n//Shadow settings\nvar shadowBlur: CGFloat\nvar shadowOpacity: Float\nvar shadowColor: UIColor\nvar backgroundImage: UIImage?\nvar backgroundColor: UIColor\n\nvar textColor: UIColor \t//Color used for the labels\nvar insets: CGFloat \t//Spacing between content and card borders\nvar cardRadius: CGFloat //Corner radius of the card\nvar icons: [UIImage]? \t//DataSource for CardGroupSliding\nvar blurEffect: UIBlurEffectStyle //Blur effect of CardGroup\n```\n\n## Usage\n\n### CardPlayer\n```swift\nlet card = CardPlayer(frame: CGRect(x: 40, y: 50, width: 300 , height: 360))\ncard.textColor = UIColor.black\ncard.videoSource = URL(string: \"http://clips.vorwaerts-gmbh.de/big_buck_bunny.mp4\")\nself.addChildViewController(card.player) /// IMPORTANT: Don't forget this\n \ncard.playerCover = UIImage(named: \"mvBackground\")! // Shows while the player is loading\ncard.playImage = UIImage(named: \"CardPlayerPlayIcon\")! // Play button icon\n \ncard.isAutoplayEnabled = true\ncard.shouldRestartVideoWhenPlaybackEnds = true\n \ncard.title = \"Big Buck Bunny\"\ncard.subtitle = \"Inside the extraordinary world of Buck Bunny\"\ncard.category = \"today's movie\"\n \nview.addSubview(card)\n```\n\n### CardGroupSliding\n```swift\n let icons: [UIImage] = [ \n \n UIImage(named: \"grBackground\")!,\n UIImage(named: \"background\")!,\n UIImage(named: \"flappy\")!,\n UIImage(named: \"flBackground\")!,\n UIImage(named: \"icon\")!,\n UIImage(named: \"mvBackground\")!\n \n ] // Data source for CardGroupSliding\n \n let card = CardGroupSliding(frame: CGRect(x: 40, y: 50, width: 300 , height: 360))\n card.textColor = UIColor.black\n \n card.icons = icons\n card.iconsSize = 60\n card.iconsRadius = 30\n \n card.title = \"from the editors\"\n card.subtitle = \"Welcome to XI Cards !\"\n\n view.addSubview(card)\n```\n\n## Thanksto\n\n- **Patrick Piemonte** - providing [Player](https://github.com/piemonte/Player) framework used in [CardPlayer.swift](https://raw.githubusercontent.com/PaoloCuscela/Cards/master/Cards/CardPlayer.swift)\n\n## License\n\nCards is released under the [MIT License](LICENSE).", |
| "homepage": "https://github.com/PaoloCuscela/Cards", |
| "Paolo Cuscela": "cuscio.2@gmail.com" |
| "git": "https://github.com/PaoloCuscela/Cards.git", |
| "source_files": "Cards/*", |
| "pushed_with_swift_version": "4.0" |